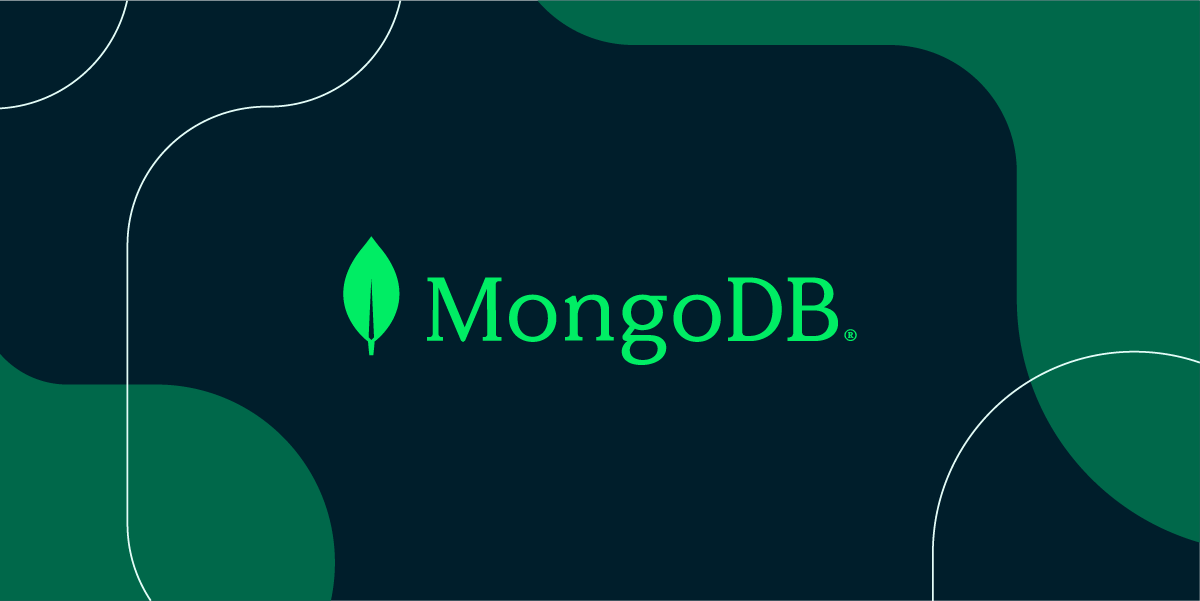
CRUD operations in MongoDB Shell
Introduction
Assuming that the database is already created in the mongoDB shell. We can perform CRUD operations like create, read, update, and delete documents. A document is basically a record in MongoDB. It is a data structure composed of field and value pairs. MongoDB documents are similar to JSON objects. The values of fields may include other documents, arrays, and arrays of documents.
Prerequisites
- Install mongodb-community
- Refer to the blog Create database in MongoDB for installing using brew and connect to the MongoDB Shell
Create database command
Execute the following command to create a database with name sample_db
> use sample_db
CRUD Operations
Insert a single Document
To insert a single document in the collection, execute db.collection.insertOne()
Note: If the -id
field is not specifies then MongoDb adds the id field with ObjectId value to the document inserted.
db.users.insertOne({first-name: "Anchal", last-name:"Gupta", age: 24})
Insert Multiple Documents
We can insert multiple documents in the collection by using db.collection.insertMany()
command.
db.departments.insertMany([ { name: "sales", people: 10, }, { name: "backend", people: 15, }, ]);
Read All Documents in a Collection
To read all the documents (data) in a collection, execute db.collection.find()
It will return all the documents inside a collection.
Read documents by specifying field and value
To read any specific document, we can specify the field and value with the command db.document.find({"field": "value"})
It will return the documents that match the specifications.
We can also specify query filters and add more specifications
Example: The following query will return upto 5 users of age greater than 18.
db.users.find( {age: {$gt: 18} } ).limit(5)
Update single document
Update commands are used to modify the existing documents in a collection.
To update a document in the collection, execute db.collection.updateOne()
along with the update operators such as $set
to modify field values.
db.departments.updateOne( { name:"sales", }, { $set: { people:20, } })
The first document where name
equals sales
will be updated.
Update multiple document
To update multiple document, execute the command db.departments.updateMany()
.
db.departments.updateMany( {people: {$gt:10}, { $set:{location:"tokyo"}, })
It will update all the documents where people are greater than 10
Replace a document
To replace entire content of the document expect the _id
field, pass the new document as the second argument and execute the command db.departments.replaceOne()
.
While executing the replace command, do not include the update operators expression.
db.departments.replaceOne( {name:"sales"}, {name:"design", people:8} )
It will replace the document where name is equal to the sales with the new document {name:"design", people:8}
passed as the second arguement
Delete one document
To delete a document from the collection, execute the command db.collection.deleteOne()
.
db.departments.deleteOne({name:"design"})
It will delete the first document where the name
is equal to design
Delete documents that match the condition
To delete multiple documents from the collection, execute the command db.collection.deleteMany()
.
db.departments.deleteMany({location:"tokyo"})
It will delete all the documents from the collection where location
is equal to tokyo
Delete all documents
To delete all the documents from the collection, execute the command db.departments.deleteMany({})
Conclusion
I tried executing the MongoDB crud operations (create, read, update, delete) using the MongoDB shell. CRUD operations describe the conventions of a user-interface that let users view, search, and modify parts of the database.